Selecting Appropriate Collection in Java
When it comes to working with data in Java, selecting the appropriate collection is akin to choosing the right tool for the job. Java offers a plethora of collection classes, each designed for specific use cases and scenarios. In this comprehensive guide, we’ll explore the world of Java collections, providing insights, best practices, and code examples to help you make informed decisions when selecting the ideal collection for your needs.
Understanding the Java Collection Framework
Before diving into the specifics of choosing collections, let’s briefly review the Java Collection Framework. The framework is a well-organized hierarchy of interfaces and classes for handling and storing collections of objects. It provides a unified and efficient way to work with data structures, making it an essential part of Java programming.
The core interfaces in the Java Collection Framework include:
-
Collection
: The root interface that defines the basic methods and behaviors common to all collection types. -
List
: An ordered collection that allows duplicate elements. Elements can be accessed by their index. -
Set
: An unordered collection that does not allow duplicate elements. -
Map
: A collection of key-value pairs, where each key is associated with a value.
Now, let’s delve into the factors to consider when choosing the right collection type for your Java project.
Quick Flowchart
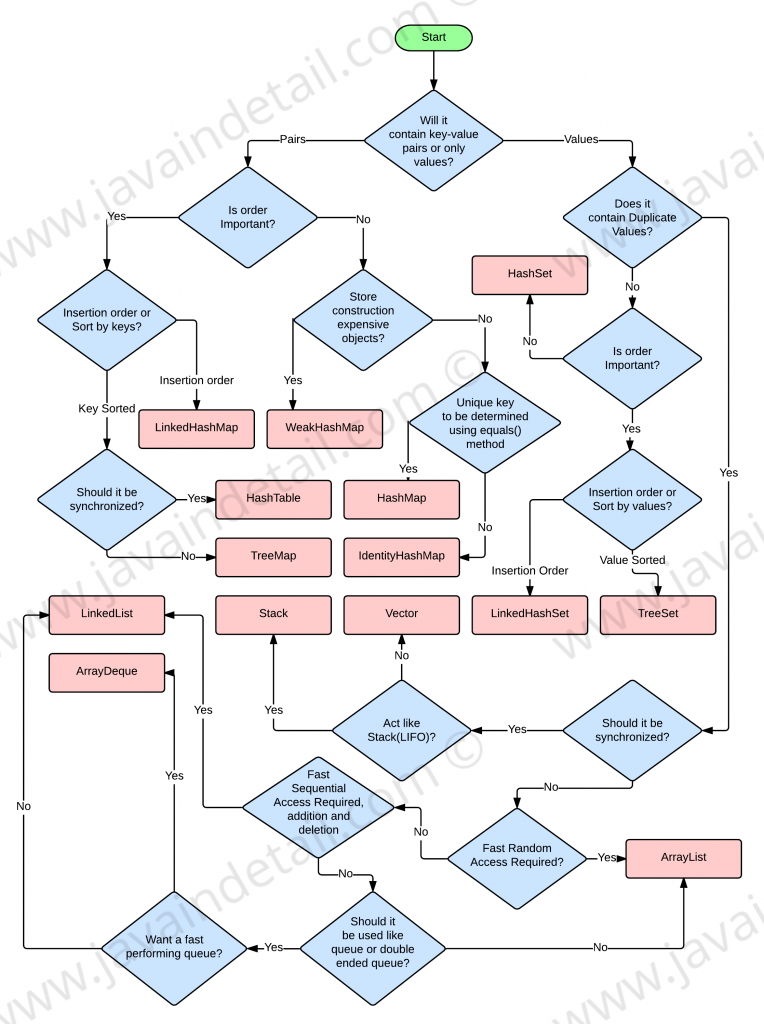
Factors to Consider
1. Data Retrieval and Search Efficiency
Different collection types provide varying levels of efficiency when it comes to data retrieval and search operations. If your application frequently requires fast access to elements by their index (position), ArrayList
or LinkedList
may be suitable choices. On the other hand, if you need to quickly search for specific elements, HashSet
or HashMap
may offer better performance due to their hashing mechanisms.
Example - ArrayList vs. LinkedList for Data Retrieval:
List<String> arrayList = new ArrayList<>();
List<String> linkedList = new LinkedList<>();
// Add elements
arrayList.add("Apple");
linkedList.add("Apple");
// Access elements by index
String fromArrayList = arrayList.get(0); // O(1)
String fromLinkedList = linkedList.get(0); // O(n) for LinkedList
2. Duplicate Elements
Consider whether your collection should allow duplicate elements or not. If duplicates are not permissible, opt for Set
implementations such as HashSet
or TreeSet
. If duplicates are allowed and you need to maintain the order of insertion, List
implementations like ArrayList
or LinkedList
are appropriate.
Example - HashSet vs. ArrayList for Handling Duplicates:
Set<String> uniqueNames = new HashSet<>();
List<String> namesWithDuplicates = new ArrayList<>();
// Adding elements
uniqueNames.add("Alice");
uniqueNames.add("Bob");
uniqueNames.add("Alice"); // Ignored, no duplicates allowed
namesWithDuplicates.add("Alice");
namesWithDuplicates.add("Bob");
namesWithDuplicates.add("Alice"); // Allowed
3. Sorting Requirements
If your data needs to be sorted, you should choose a collection that supports sorting. TreeSet
and TreeMap
are sorted implementations of Set
and Map
, respectively, and automatically arrange elements in natural order or according to a custom comparator.
Example - Sorting with TreeSet:
Set<Integer> sortedSet = new TreeSet<>();
sortedSet.add(3);
sortedSet.add(1);
sortedSet.add(2);
// Elements are automatically sorted
System.out.println(sortedSet); // Output: [1, 2, 3]
4. Key-Value Pairs
For scenarios where you need to associate values with keys, Map
implementations such as HashMap
and TreeMap
are the go-to choices. These collections allow you to store and retrieve values using unique keys, offering fast key-based access.
Example - Using HashMap for Key-Value Mapping:
Map<String, Integer> ageMap = new HashMap<>();
// Adding key-value pairs
ageMap.put("Alice", 25);
ageMap.put("Bob", 30);
// Retrieving values by key
int aliceAge = ageMap.get("Alice"); // Returns 25
5. Thread Safety
Consider whether your collection needs to be thread-safe. In a multi-threaded environment, certain collection types like ArrayList
and HashMap
are not thread-safe by default. To ensure thread safety, you can use synchronized collections or specialized thread-safe implementations like ConcurrentHashMap
or CopyOnWriteArrayList
.
Example - Using ConcurrentHashMap for Thread Safety:
Map<String, Integer> threadSafeAgeMap = new ConcurrentHashMap<>();
threadSafeAgeMap.put("Alice", 25);
threadSafeAgeMap.put("Bob", 30);
// Thread-safe operations
int aliceAge = threadSafeAgeMap.get("Alice"); // Safe in a multi-threaded environment
6. Memory Usage
Different collection types have varying memory usage characteristics. For large datasets where memory efficiency is crucial, consider collections like HashSet
and HashMap
that rely on hashing mechanisms and typically use less memory than their ordered counterparts like TreeSet
and TreeMap
.
Example - Memory Efficiency of HashSet vs. TreeSet:
Set<Integer> hashSet = new HashSet<>();
Set<Integer> treeSet = new TreeSet<>();
for (int i = 0; i < 10000; i++) {
hashSet.add(i);
treeSet.add(i);
}
// HashSet consumes less memory due to hashing
Common Java Collection Types
Let’s explore some of the most commonly used Java collection types and their characteristics:
1. ArrayList
- Implements the
List
interface. - Allows duplicate elements and maintains insertion order.
- Provides fast random access by index.
- Suitable for scenarios where elements need to be accessed frequently but not frequently inserted or removed.
Example - Using ArrayList:
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
String first = names.get(0); // Access by index
2. HashSet
- Implements the
Set
interface. - Does not allow duplicate elements.
- Offers constant-time performance for basic operations (add, remove, contains) on average.
- Suitable for scenarios where uniqueness is important and order doesn’t matter.
Example - Using HashSet:
Set<Integer> numbers = new HashSet<>();
numbers.add(1);
numbers.add(2);
numbers.add(3);
boolean containsTwo = numbers.contains(2); // Returns true
3. HashMap
- Implements the
Map
interface. - Stores key-value pairs.
- Provides fast key-based access and efficient insertion and removal.
- Suitable for mapping keys to values in a non-sorted manner.
Example - Using HashMap:
Map<String, Integer> ageMap = new HashMap<>();
ageMap.put("Alice", 25);
ageMap.put("Bob", 30);
int aliceAge = ageMap.get("Alice"); // Returns 25
4. TreeSet
- Implements the
Set
interface. - Stores elements in sorted order.
- Suitable for scenarios where elements need to be stored in a specific order.
- Offers efficient sorted set operations.
Example - Using TreeSet:
Set<String> sortedNames = new TreeSet<>();
sorted
Names.add("Bob");
sortedNames.add("Alice");
sortedNames.add("Charlie");
String first = sortedNames.first(); // Returns "Alice"
5. LinkedList
- Implements the
List
interface. - Efficient for inserting and removing elements in the middle of the list.
- Offers slower random access compared to
ArrayList
. - Suitable for scenarios where frequent insertion and deletion are required.
Example - Using LinkedList:
List<String> names = new LinkedList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
String first = names.get(0); // Access by index
Enjoy Reading This Article?
Here are some more articles you might like to read next: